반응형
1. 노션에 들어가면 안보이는 새로운 글자가 보입니다.
AI로 글쓰기 시작
스페이스 바를 누르면 AI기능이 나옵니다.
2. AI에게 요청할 메뉴를 고를 수 있습니다.
더 보기를 누르면 더 많은 메뉴를 볼 수 있습니다.
3. 파이썬에게 벽돌깨기 소스를 물어 봤습니다.
4. 결과 ① - 아래는 파이썬으로 만들어진 벽돌깨기 게임 소스코드입니다.
import pygame
import sys
import math
import random
# Initialize pygame
pygame.init()
# Set screen dimensions
screen_width = 640
screen_height = 480
# Create the screen
screen = pygame.display.set_mode((screen_width, screen_height))
# Set the clock
clock = pygame.time.Clock()
# Set font
font = pygame.font.Font(None, 36)
# Set colors
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# Set game variables
ball_speed = 5
ball_x = screen_width / 2
ball_y = screen_height / 2
ball_direction = random.randint(0, 360)
ball_radius = 10
ball_color = BLUE
paddle_width = 100
paddle_height = 20
paddle_x = (screen_width - paddle_width) / 2
paddle_y = screen_height - paddle_height - 10
paddle_color = GREEN
brick_width = 60
brick_height = 20
brick_color = RED
brick_padding = 10
brick_offset_top = 50
brick_offset_left = 10
# Create bricks
bricks = []
for i in range(5):
brick_row = []
for j in range(8):
brick_x = j * (brick_width + brick_padding) + brick_offset_left
brick_y = i * (brick_height + brick_padding) + brick_offset_top
brick_rect = pygame.Rect(brick_x, brick_y, brick_width, brick_height)
brick_row.append(brick_rect)
bricks.append(brick_row)
# Initialize the score
score = 0
# Game loop
while True:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# Move the paddle
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle_x > 0:
paddle_x -= 5
if keys[pygame.K_RIGHT] and paddle_x < screen_width - paddle_width:
paddle_x += 5
# Move the ball
ball_dx = ball_speed * math.cos(ball_direction)
ball_dy = ball_speed * math.sin(ball_direction)
ball_x += ball_dx
ball_y += ball_dy
# Bounce the ball off the walls
if ball_x < ball_radius or ball_x > screen_width - ball_radius:
ball_direction = math.pi - ball_direction
if ball_y < ball_radius:
ball_direction = -ball_direction
if ball_y > screen_height - ball_radius:
ball_y = screen_height - ball_radius
ball_direction = -ball_direction
# Bounce the ball off the paddle
if ball_y > paddle_y - ball_radius and ball_x > paddle_x and ball_x < paddle_x + paddle_width:
ball_direction = -ball_direction
# Check for collisions with bricks
for i in range(len(bricks)):
for j in range(len(bricks[i])):
if ball.colliderect(bricks[i][j]):
brick = bricks[i][j]
cx = ball.x - brick.x
cy = ball.y - brick.y
if abs(cx) > abs(cy):
ball_direction = math.pi - ball_direction
else:
ball_direction = -ball_direction
bricks[i][j] = None
score += 10
# Draw the screen
screen.fill(BLACK)
pygame.draw.circle(screen, ball_color, (int(ball_x), int(ball_y)), ball_radius)
pygame.draw.rect(screen, paddle_color, (int(paddle_x), int(paddle_y), int(paddle_width), int(paddle_height)))
for i in range(len(bricks)):
for j in range(len(bricks[i])):
if bricks[i][j]:
pygame.draw.rect(screen, brick_color, bricks[i][j])
score_text = font.render(f"Score: {score}", True, WHITE)
screen.blit(score_text, (10, 10))
pygame.display.flip()
# Set the frame rate
clock.tick(60)
4. 결과 ② - 똑같이 물어 봤는데, 다른 결과의 소스가 나왔습니다.
import pygame
import random
# 게임 창 설정
pygame.init()
screen_width = 480
screen_height = 640
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("벽돌깨기")
# FPS
clock = pygame.time.Clock()
# 이미지 불러오기
background = pygame.image.load("background.png")
stage = pygame.image.load("stage.png")
stage_size = stage.get_rect().size
stage_height = stage_size[1]
character = pygame.image.load("character.png")
character_size = character.get_rect().size
character_width = character_size[0]
character_height = character_size[1]
character_x_pos = (screen_width / 2) - (character_width / 2)
character_y_pos = screen_height - stage_height - character_height
# 이동할 좌표
to_x = 0
character_speed = 5
# 무기 불러오기
weapon = pygame.image.load("weapon.png")
weapon_size = weapon.get_rect().size
weapon_width = weapon_size[0]
weapons = []
weapon_speed = 10
# 공 불러오기
ball_images = [
pygame.image.load("ball1.png"),
pygame.image.load("ball2.png"),
pygame.image.load("ball3.png"),
pygame.image.load("ball4.png")
]
ball_speed_y = [-18, -15, -12, -9]
balls = []
ball_sizes = []
for ball_image in ball_images:
ball_size = ball_image.get_rect().size
ball_sizes.append(ball_size)
max_ball_count = 20
# 공과 무기 충돌 처리
def check_collision(character_x_pos, character_y_pos, weapons, balls):
for ball_index, ball in enumerate(balls):
ball_pos_x = ball[0]
ball_pos_y = ball[1]
ball_size_x = ball_sizes[ball[2]][0]
ball_size_y = ball_sizes[ball[2]][1]
if character_x_pos < ball_pos_x + ball_size_x and character_x_pos + character_width > ball_pos_x and character_y_pos < ball_pos_y + ball_size_y and character_y_pos + character_height > ball_pos_y:
return True
for weapon_index, weapon in enumerate(weapons):
weapon_pos_x = weapon[0]
weapon_pos_y = weapon[1]
if weapon_pos_x < ball_pos_x + ball_size_x and weapon_pos_x + weapon_width > ball_pos_x and weapon_pos_y < ball_pos_y + ball_size_y and weapon_pos_y + weapon_size[1] > ball_pos_y:
del weapons[weapon_index]
del balls[ball_index]
break
return False
# 게임 실행
running = True
while running:
dt = clock.tick(60)
# 이벤트 처리
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
to_x -= character_speed
elif event.key == pygame.K_RIGHT:
to_x += character_speed
elif event.key == pygame.K_SPACE:
weapon_x_pos = character_x_pos + (character_width / 2) - (weapon_width / 2)
weapon_y_pos = character_y_pos
weapons.append([weapon_x_pos, weapon_y_pos])
if event.type == pygame.KEYUP:
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
to_x = 0
# 캐릭터 이동
character_x_pos += to_x
if character_x_pos < 0:
character_x_pos = 0
elif character_x_pos > screen_width - character_width:
character_x_pos = screen_width - character_width
# 무기 이동
weapons = [[w[0], w[1] - weapon_speed] for w in weapons]
# 천장에 닿은 무기 삭제
weapons = [[w[0], w[1]] for w in weapons if w[1] > 0]
# 공 생성
if len(balls) < max_ball_count:
ball_pos_x = random.randint(0, screen_width - ball_sizes[0][0])
ball_pos_y = 0
ball_speed_x = random.randint(2, 10)
ball_speed_y = ball_speed_y[random.randint(0, 3)]
ball_image_index = random.randint(0, 3)
balls.append([ball_pos_x, ball_pos_y, ball_image_index, ball_speed_x, ball_speed_y])
# 공 이동
for ball_index, ball in enumerate(balls):
ball_pos_x = ball[0]
ball_pos_y = ball[1]
ball_speed_x = ball[3]
ball_speed_y = ball[4]
if ball_pos_x < 0 or ball_pos_x > screen_width - ball_sizes[ball[2]][0]:
ball[3] = -ball_speed_x
elif ball_pos_y > screen_height - stage_height - ball_sizes[ball[2]][1]:
ball[4] = -ball_speed_y
else:
ball[0] += ball_speed_x * dt / 30
ball[1] += ball_speed_y * dt / 30
# 충돌 처리
if check_collision(character_x_pos, character_y_pos, weapons, balls):
running = False
# 화면에 그리기
screen.blit(background, (0, 0))
for weapon_x_pos, weapon_y_pos in weapons:
screen.blit(weapon, (weapon_x_pos, weapon_y_pos))
for ball in balls:
screen.blit(ball_images[ball[2]], (ball[0], ball[1]))
screen.blit(stage, (0, screen_height - stage_height))
screen.blit(character, (character_x_pos, character_y_pos))
pygame.display.update()
# 게임 종료
pygame.quit()
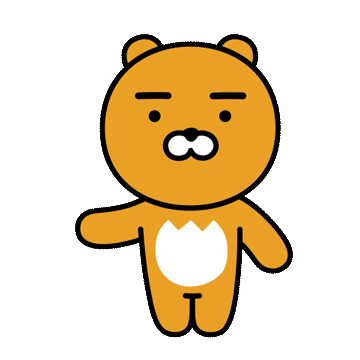
이상 노션의 AI를 이용한 파이썬 벽돌깨기 소스 끝~~~!!!